详情请点阅读全文
React生命周期函数:
【官方文档】:https://reactjs.org/docs/react-component.html
【定义】组件加载之前,组件加载完成,以及组件更新数据,组件销毁。 触发的一系列的方法 ,这就是组件的生命周期函数
一、组件加载的时候触发的函数:
constructor 、componentWillMount、 render 、componentDidMount
组件加载触发函数实例
【Lifecycle.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| import React, { Component } from 'react';
class Lifecycle extends Component { constructor(props){ console.log('01构造函数');
super(props); this.state={ title:'生命同期示例组件' } }
componentWillMount(){ console.log('02组件将要挂载'); }
componentDidMount(){ console.log('04组件将要挂载'); }
render() { console.log('03数据渲染render'); return ( <div> <h1>生命同期函数:加载时触发的函数</h1> <br/><hr/>
</div> ); } } export default Lifecycle;
|
【Home.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| import React, { Component } from 'react'; import Lifecycle from './Lifecycle';
class Home extends Component { constructor(props){ super(props); this.state={ title:'生命同期函数示例' } }
render() { return ( <div> <Lifecycle /> </div> ); } } export default Home;
|
【App.js】
1 2 3 4 5 6 7 8 9 10 11 12 13
| import React from 'react'; import './App.css'; import Home from './components/Home.js';
function App() { return ( <div className="App"> <Home /> <hr/> </div> ); } export default App;
|
【结果】刷新页面,函数将逐个被加载(警告部分不用管它)
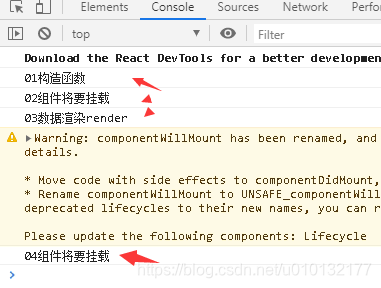
二、组件数据更新的时候触发的生命周期函数:
shouldComponentUpdate、componentWillUpdate、render、componentDidUpdate
【Lifecycle.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| import React, { Component } from 'react';
class Lifecycle extends Component { constructor(props){ super(props); this.state={ title:'生命同期示例组件', msg:'没更新数据之前消息' } }
shouldComponentUpdate(nextProps, nextState){ console.log('01是否要更新数据'); console.log(nextProps); console.log(nextState); return true; }
componentWillUpdate(){ console.log('02组件将要更新'); } componentDidUpdate(){ console.log('04组件数据更新完成'); }
setMsg=()=>{ this.setState({ msg:'我是改变后的msg的数据' }) }
render() { console.log('03数据渲染render'); return ( <div> <h1>生命同期函数:更新数据时触发的函数</h1> <h2>{this.state.msg}</h2> <button onClick={this.setMsg}>更新state数据</button> <br/><hr/> </div> ); } } export default Lifecycle;
|
【Home.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| import React, { Component } from 'react'; import Lifecycle from './Lifecycle';
class Home extends Component { constructor(props){ super(props); this.state={ title:'生命同期函数示例' } }
render() { return ( <div> <Lifecycle /> </div> ); } } export default Home;
|
【App.js】
1 2 3 4 5 6 7 8 9 10 11 12
| import React from 'react'; import './App.css'; import Home from './components/Home.js';
function App() { return ( <div className="App"> <Home /> </div> ); } export default App;
|
【结果】点按键后,按顺序进行数据更新:
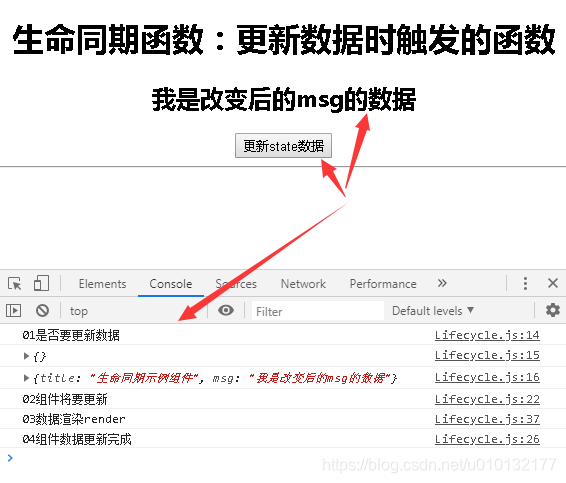
【注】console其中第2、3条数据来自[Lifecycle.js]的【console.log(nextProps); console.log(nextState);】
将在下一主题讲到:三、
三、你在父组件里面改变props传值的时候触发的:
componentWillReceiveProps
【Lifecycle.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| import React, { Component } from 'react';
class Lifecycle extends Component { constructor(props){ super(props); this.state={ title:'生命同期示例组件', msg:'没更新数据之前消息' } }
componentWillReceiveProps(){ console.log('00父子组件传值,父组件里面改变了props的值触发的方法') }
shouldComponentUpdate(nextProps, nextState){ console.log('01是否要更新数据'); console.log(nextProps); console.log(nextState); return true; }
componentWillUpdate(){ console.log('02组件将要更新'); } componentDidUpdate(){ console.log('04组件数据更新完成'); }
render() { console.log('03数据渲染render'); return ( <div> {} <h3>{this.props.title}</h3> <br/><hr/> </div> ); } } export default Lifecycle;
|
【Home.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| import React, { Component } from 'react'; import Lifecycle from './Lifecycle'; import { thisTypeAnnotation } from '@babel/types';
class Home extends Component { constructor(props){ super(props); this.state={ title:'父组件标题:生命同期函数示例', flag:true } }
changtitle=()=>{ this.setState({ title:'改变后的父组件标题' }) }
render() { return ( <div> <h1>生命周期函数:向子组件传值</h1> { //后面的title=xxx为向子组件传值 } <Lifecycle title={this.state.title} /> <button onClick={this.changtitle}>改变state要传给子组件的值</button>
</div> ); } } export default Home;
|
【App.js】
1 2 3 4 5 6 7 8 9 10 11 12
| import React from 'react'; import './App.css'; import Home from './components/Home.js';
function App() { return ( <div className="App"> <Home /> </div> ); } export default App;
|
【结果】:
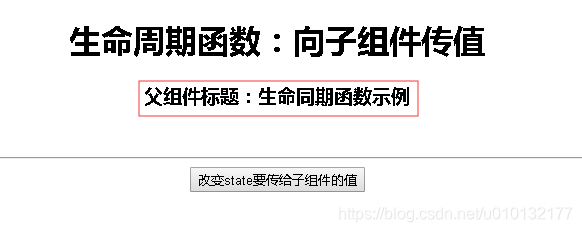
点改变之后:
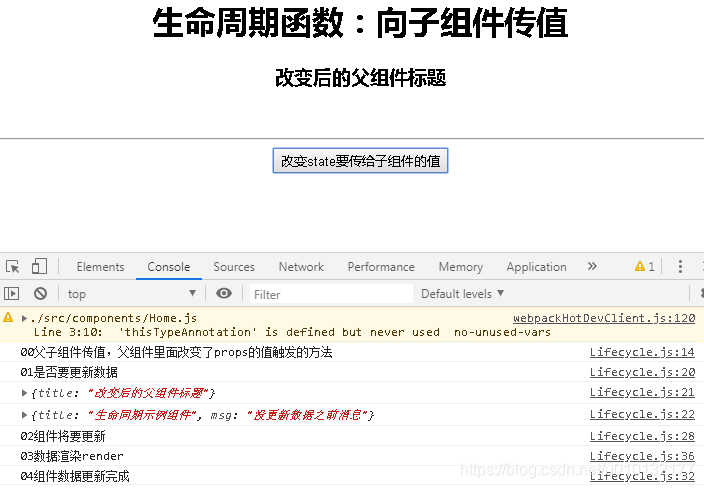
四、组件销毁的时候触发的:
componentWillUnmount
【应用场景】:比如编写文件时,放弃保存,就要销毁那个页面的组件;
【Lifecycle.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| import React, { Component } from 'react';
class Lifecycle extends Component { constructor(props){ super(props); this.state={ title:'生命同期示例组件', msg:'没更新数据之前消息' } }
componentWillUnmount(){ console.log('组件已销毁'); }
setMsg=()=>{ this.setState({ msg:'我是改变后的msg的数据' }) }
render() { console.log('03数据渲染render'); return ( <div> <h2>{this.state.msg}</h2> <button onClick={this.setMsg}>更新state数据</button> <br/><hr/> </div> ); } } export default Lifecycle;
|
【Home.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| import React, { Component } from 'react'; import Lifecycle from './Lifecycle';
class Home extends Component { constructor(props){ super(props); this.state={ title:'生命同期函数示例', flag:true } }
setFlag=()=>{ this.setState({ flag:!this.state.flag }) }
render() { return ( <div> <h1>销毁组件生命周期函数示例</h1> { //【是否销毁子组件重点代码】:如果flag=true加载<Lifecycle />组件,否则加载空; this.state.flag?<Lifecycle />:"" } <br/> <button onClick={this.setFlag}>销毁/加载子组件</button>
</div> ); } } export default Home;
|
【App.js】
1 2 3 4 5 6 7 8 9 10 11 12
| import React from 'react'; import './App.css'; import Home from './components/Home.js';
function App() { return ( <div className="App"> <Home /> </div> ); } export default App;
|
【结果】:
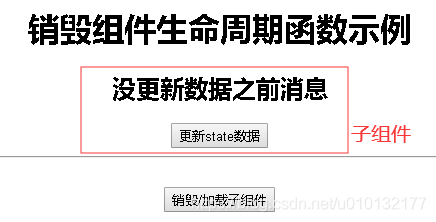
【点销毁后】:
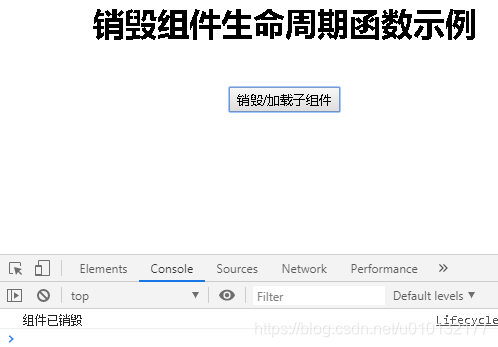
五、【总结】必须记住的生命周期函数:
*加载的时候:componentWillMount、 render 、componentDidMount(dom操作要放此处)
更新的时候:componentWillUpdate、render、componentDidUpdate
*销毁的时候: componentWillUnmount