详情请点阅读全文
概述
新闻列表 —跳转—> 详情页 时,想把列表对应的id传到详情页里,可用到三种传值方法:
1、动态路由传值
2、get传值
3、localstorage传值
一、动态路由传值
【App.js】主要路由配置都在此处。01所在
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| import React from 'react'; import './App.css';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom'; import Home from './components/Home'; import News from './components/News'; import Product from './components/Product'; import Content from './components/Content';
function App() { return ( <Router> <div> <header className="title"> <Link to="/">首页</Link> | <Link to="/news">新闻</Link> | <Link to="/product">商品</Link> | </header> <br /><hr />
<Route exact path="/" component={Home} /> <Route path="/news" component={News} /> <Route path="/product" component={Product} /> {/* 【01】配置动态路由 (:aid即动态路由意思) 02在News.js */} <Route path="/Content/:aid" component={Content} /> </div> </Router> ); } export default App;
|
【News.js】路由配置02步所在
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| import React, { Component } from 'react'; import {Link} from 'react-router-dom';
class News extends Component { constructor(props) { super(props); this.state = { list:[ { aid:1, title:'新闻列表111' }, { aid:2, title:'新闻列表222' }, { aid:3, title:'新闻列表333' }, { aid:4, title:'新闻列表444' }, { aid:5, title:'新闻列表555' }, ] }; } render() { return ( <div> <h1>我是新闻组件</h1> <ul> {this.state.list.map((value,key)=>{ //【02】动态路由配置,获取对应值的aid值传到链接里。地址栏会变成类似 localhost:3000/content/1 return <li key={key}><Link to={`/Content/${value.aid}`}>{value.title}</Link></li> }) } </ul> </div> ); } } export default News;
|
【Content.js】路由配置03步所在
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import React, { Component } from 'react';
class Content extends Component { constructor(props) { super(props); this.state = { }; } componentDidMount(){ console.log(this.props) console.log(this.props.match.params.aid) } render() { return ( <div> 我是新闻详情组件,当前aid值为:{this.props.match.params.aid} </div> ); } } export default Content;
|
【效果】在新闻列表页点aid=2项:
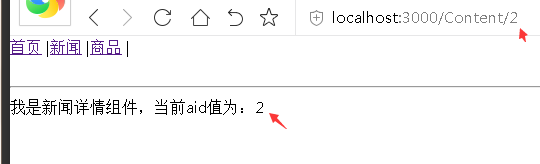
二、get传值
get传值主要特征是地址有个 ?xxx:http://localhost:3000/ProductDetail?aid=1
【App.js】路由配置同路由
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| import React from 'react'; import './App.css';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom'; import Home from './components/Home'; import News from './components/News'; import Content from './components/Content';
import Product from './components/Product'; import ProductDetail from './components/ProductDetail';
function App() { return ( <Router> <div> <header className="title"> <Link to="/">首页</Link> | <Link to="/news">新闻</Link> | <Link to="/product">商品</Link> | </header>
<br /><hr />
<Route exact path="/" component={Home} /> <Route path="/news" component={News} /> <Route path="/Content/:aid" component={Content} />
<Route path="/product" component={Product} /> {/* 【01】get配置 02在Product.js */} <Route path="/ProductDetail" component={ProductDetail} /> </div> </Router> ); } export default App;
|
【Product.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| import React, { Component } from 'react'; import {Link} from 'react-router-dom';
class Product extends Component { constructor(props) { super(props); this.state = { list:[ { aid:1, title:'商品列表111' }, { aid:2, title:'商品列表222' }, { aid:3, title:'商品列表333' }, { aid:4, title:'商品列表444' }, { aid:5, title:'商品列表555' }, ] }; } render() { return ( <div> <h1>我是商品组件</h1> <ul> {this.state.list.map((value,key)=>{ //【02】get配置:获取对应值的aid值传到链接里。地址栏会变成类似 localhost:3000/ProductDetail?aid=1 return <li key={key}><Link to={`/ProductDetail?aid=${value.aid}`}>{value.title}</Link></li> }) } </ul>
</div> ); } } export default Product;
|
【ProductDetail.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| import React, { Component } from 'react';
class ProductDetail extends Component { constructor(props) { super(props); this.state = { }; }
componentDidMount(){
console.log(this.props) console.log(this.props.location.search) } render() { return ( <div> <h1>我是商品详情组件当前商品aid是:{this.props.location.search}</h1> </div> ); } } export default ProductDetail;
|
【效果】:点 [商品列表] 任意一个,会把 [对应的aid] 通过地址栏传到 [商品详情页] 去:
问题:此处需要得到的是 5 这个值,但结果确不同,将在下一节解决
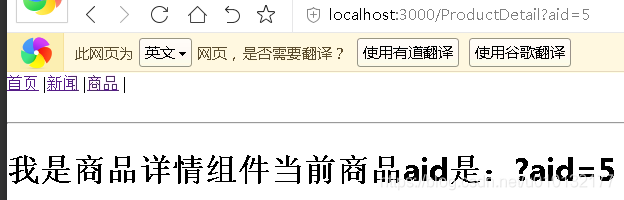
三、React中使用url模块,解析url
1.安装url模块:
2.引入页面:
url解析使用
解决:将解决上一节传过来的aid值成了:?aid=xx ,直接获取xx;
【App.js】:代码同上
【Product.js】:代码同上
【ProductDetail.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| import React, { Component } from 'react';
import url from 'url';
class ProductDetail extends Component { constructor(props) { super(props); this.state = { }; }
componentDidMount(){
console.log(url.parse(this.props.location.search,true))
var query=url.parse(this.props.location.search,true).query; console.log(query); } render() { return ( <div> <h1>我是商品详情组件当前商品</h1> </div> ); } } export default ProductDetail;
|
效果:在地址栏随意传过来的参数都会进行解析:
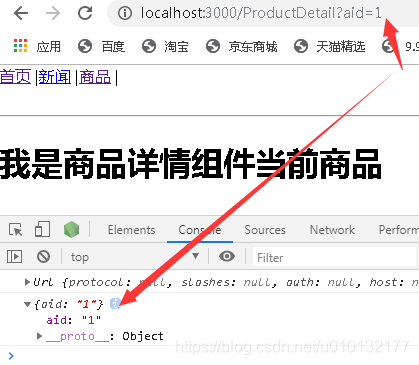
自己随便写个参数:都可进行解析
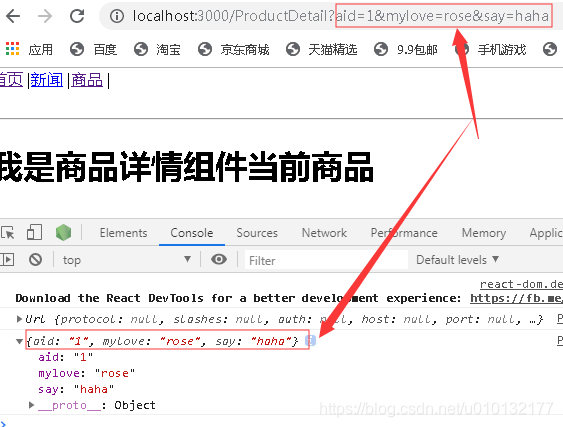
三、总结
react动态路由传值:
1、动态路由配置
<Route path="/content/:aid" component={Content} />
2、对应的动态路由加载的组件里面获取传值
this.props.match.params
跳转:<Link to={`/content/${value.aid}`}>{value.title}</Link>
react get传值:
1、获取 this.props.location.search