详情请点阅读全文
一、自写一个方法(函数),然后用按钮onClick触发它
如果想要在react模板render()内执行一个自定方法(函数),就要这样写,在components目录下新建立一个【Demo.js】:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| import React from 'react';
class Demo extends React.Component{ constructor(){ super(); this.state={ name:'xiaoming' } }
run2(){ alert('这是一个待执行函数') }
render(){ return( <div> <button onClick={this.run2}>点这里执行函数</button> </div> ); } } export default Demo;
|
【App.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import React, { Component } from 'react'; import logo from './logo.svg';
import Demo from './components/Demo.js';
class App extends Component { render (){ return( <div className="App"> <h1>这里是app.js根组件</h1>
<Demo /> </div> );} } export default App;
|
进入项目根目录:运行cmd:npm start,运行结果:
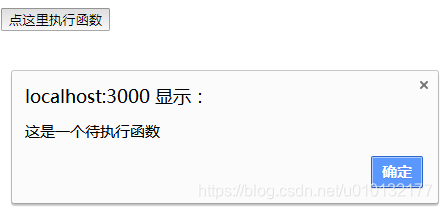
二、自写方法引用构造函数内的数据(改变this指向的)写法
写法1【Demo.js】:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| import React from 'react';
class Demo extends React.Component{ constructor(){ super();
this.state={ msg:'this is a state messages!' } }
run2(){ alert(this.state.msg) }
render(){ return( <div> <button onClick={this.run2.bind(this)}>点这里执行函数</button> </div> ); } }
export default Demo;
|
写法2【Demo.js】:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| import React from 'react';
class Demo extends React.Component{ constructor(){ super(); this.state={ msg:'方法2 成功获取到我咯——state信息!' }
this.getmsg=this.getmsg.bind(this); }
getmsg(){ alert(this.state.msg) }
render(){ return( <div> <button onClick={this.getmsg}>点这里执行函数</button> </div> ); } } export default Demo;
|
写法3:【Demo.js】最省事写法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import React from 'react';
class Demo extends React.Component{ constructor(){ super(); this.state={ msg:'方法3 成功获取到我咯——state信息!' } }
getmsg=()=>{ alert(this.state.msg) } render(){ return( <div> <button onClick={this.getmsg}>点这里执行函数</button> </div> ); } } export default Demo;
|
不用.bind(this)时报错,因为它无法正确指向
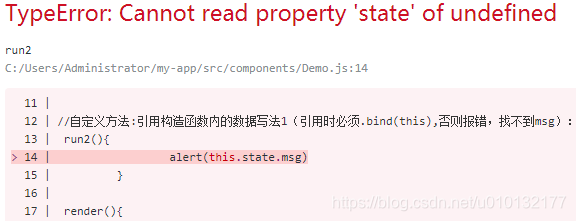
App.js用不变,直接刷新网址查看效果:
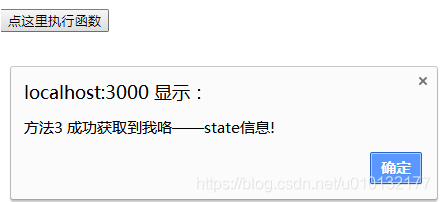
三、改变state值的写法this.setState()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| import React from 'react';
class Demo extends React.Component{ constructor(){ super(); this.state={ msg:'这是state的msg原始值' } }
changeMsg=()=>{ this.setState({ msg:"!!!!!!这是state的msg改变值!!!!!" }) }
render(){ return( <div> <h1>{this.state.msg}</h1> <button onClick={this.changeMsg}>点这里改变state值</button> </div> ); } } export default Demo;
|
运行结果:
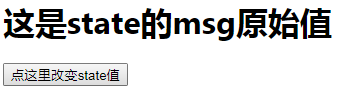
点击后:
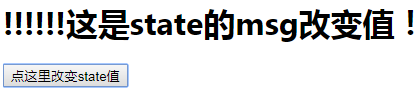
四、通过自写方法的参数,向state内传值
【1】pushMsg=(name)=>{this.setState({ msg:name})
【2】onClick={this.pushMsg.bind(this,’我是用参数传过来的值’)}
4.1传一个参数【Demo.js】:
import React from ‘react’;
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Demo extends React.Component{ constructor(props){ super(props); this.state={ msg:'原始值' } } pushMsg=(name)=>{ this.setState({ msg:name }) }
render(){ return( <div> <h1>{this.state.msg}</h1> { //【2】调用自写函数用参数传值到state } <button onClick={this.pushMsg.bind(this,'我是用参数传过来的值')}>点这里用方法传值</button> </div> ) } } export default Demo;
|
App.js:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| import React from 'react'; import logo from './logo.svg'; import './App.css'; import Demo from './components/Demo'
function App() { return ( <div className="App"> <img src={logo} className="App-logo" alt="logo" /> <Demo /> </div> ); } export default App;
|
【效果】
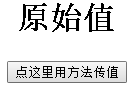
点后:
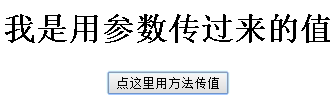
4.2通过自写函数传多个参数值到state
【 Demo.js】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| import React from 'react';
class Demo extends React.Component{ constructor(props){ super(props); this.state={ msg:'原始值', msg2:'原始值2号' } } pushMsg=(name,name2)=>{ this.setState({ msg:name, msg2:name2 }) }
render(){ return( <div> <h1>{this.state.msg}</h1> <h1>{this.state.msg2}</h1> { } <button onClick={this.pushMsg.bind(this,'我是用参数传过来的值。','传过来第二个值')}>点这里用方法传值</button> </div> ) } } export default Demo;
|
App.js不变
效果:
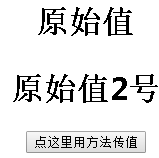
点后:
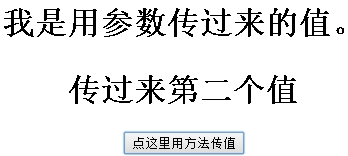